Object that handles loading/storing a PlannerData object to/from a binary stream. Serialization of vertices and edges is performed using the Boost archive method serialize. Derived vertex/edge classes are handled, presuming those classes implement the serialize method. More...
#include <ompl/control/PlannerDataStorage.h>
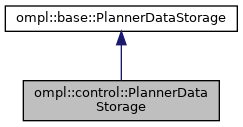
Public Member Functions | |
PlannerDataStorage ()=default | |
Default constructor. | |
~PlannerDataStorage () override=default | |
Destructor. | |
bool | load (const char *filename, base::PlannerData &pd) override |
Load the PlannerData structure from the given filename. | |
bool | load (std::istream &in, base::PlannerData &pd) override |
Deserializes the structure from the given stream. | |
bool | store (const base::PlannerData &pd, const char *filename) override |
Store (serialize) the structure to the given filename. The StateSpace and ControlSpace that was used to store the data must match those inside of the argument PlannerData. | |
bool | store (const base::PlannerData &pd, std::ostream &out) override |
Load the PlannerData structure from the given stream. The StateSpace and ControlSpace that was used to store the data must match those inside of the argument PlannerData. | |
![]() | |
PlannerDataStorage () | |
Default constructor. | |
Protected Member Functions | |
void | loadEdges (base::PlannerData &pd, unsigned int numEdges, boost::archive::binary_iarchive &ia) override |
Read numEdges from the binary input ia and store them as PlannerData. It is assumed that the edges can be cast to ompl::control::PlannerDataEdgeControl. | |
void | storeEdges (const base::PlannerData &pd, boost::archive::binary_oarchive &oa) override |
Serialize and store all edges in pd to the binary archive. It is assumed that the edges can be cast to ompl::control::PlannerDataEdgeControl. | |
![]() | |
virtual void | loadVertices (PlannerData &pd, unsigned int numVertices, boost::archive::binary_iarchive &ia) |
Read numVertices from the binary input ia and store them as PlannerData. | |
virtual void | storeVertices (const PlannerData &pd, boost::archive::binary_oarchive &oa) |
Serialize and store all vertices in pd to the binary archive. | |
Detailed Description
Object that handles loading/storing a PlannerData object to/from a binary stream. Serialization of vertices and edges is performed using the Boost archive method serialize. Derived vertex/edge classes are handled, presuming those classes implement the serialize method.
- Remarks
- Since the serialize method for vertices and edges is templated, it cannot be virtual. To serialize a derived class AND the base class data, a special call can be invoked inside of serialize that instructs the serializer to also serialize the base class. The derived class must also have a GUID exposed to the serializer for proper deserialization at runtime. This is performed with the BOOST_CLASS_EXPORT macro. An example of these items is given below: #include <boost/serialization/export.hpp>{// ---SNIP---template <class Archive>void serialize(Archive & ar, const unsigned int version){ar & boost::serialization::base_object<ompl::base::PlannerDataVertex>(*this);// ... (The other members of MyVertexClass)}};BOOST_CLASS_EXPORT(MyVertexClass);
It is assumed that the edges in stored/loaded PlannerData can be cast to PlannerDataEdgeControl in this class. If this is not the case, see ompl::base::PlannerDataStorage.
Definition at line 116 of file PlannerDataStorage.h.
The documentation for this class was generated from the following files:
- ompl/control/PlannerDataStorage.h
- ompl/control/src/PlannerDataStorage.cpp