This class allows for the definition of multiobjective optimal planning problems. Objectives are added to this compound object, and motion costs are computed by taking a weighted sum of the individual objective costs. More...
#include <ompl/base/OptimizationObjective.h>
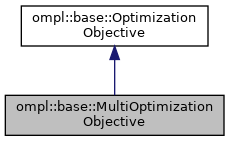
Classes | |
struct | Component |
Defines a pairing of an objective and its weight. More... | |
Public Member Functions | |
MultiOptimizationObjective (const SpaceInformationPtr &si) | |
void | addObjective (const OptimizationObjectivePtr &objective, double weight) |
Adds a new objective for this multiobjective. A weight must also be specified for specifying importance of this objective in planning. | |
std::size_t | getObjectiveCount () const |
Returns the number of objectives that make up this multiobjective. | |
const OptimizationObjectivePtr & | getObjective (unsigned int idx) const |
Returns a specific objective from this multiobjective, where the individual objectives are in order of addition to the multiobjective, and idx is the zero-based index into this ordering. | |
double | getObjectiveWeight (unsigned int idx) const |
Returns the weighing factor of a specific objective. | |
void | setObjectiveWeight (unsigned int idx, double weight) |
Sets the weighing factor of a specific objective. | |
void | lock () |
This method "freezes" this multiobjective so that no more objectives can be added to it. | |
bool | isLocked () const |
Returns whether this multiobjective has been locked from adding further objectives. | |
Cost | stateCost (const State *s) const override |
Cost | motionCost (const State *s1, const State *s2) const override |
![]() | |
OptimizationObjective (const OptimizationObjective &)=delete | |
OptimizationObjective & | operator= (const OptimizationObjective &)=delete |
OptimizationObjective (SpaceInformationPtr si) | |
Constructor. The objective must always know the space information it is part of. The cost threshold for objective satisfaction defaults to 0.0. | |
const std::string & | getDescription () const |
Get the description of this optimization objective. | |
virtual bool | isSatisfied (Cost c) const |
Check if the the given cost c satisfies the specified cost objective, defined as better than the specified threshold. | |
Cost | getCostThreshold () const |
Returns the cost threshold currently being checked for objective satisfaction. | |
void | setCostThreshold (Cost c) |
Set the cost threshold for objective satisfaction. When a path is found with a cost better than the cost threshold, the objective is considered satisfied. | |
virtual bool | isCostBetterThan (Cost c1, Cost c2) const |
Check whether the the cost c1 is considered better than the cost c2. By default, this returns true if if c1 is less than c2. | |
virtual bool | isCostEquivalentTo (Cost c1, Cost c2) const |
Compare whether cost c1 and cost c2 are equivalent. By default defined as !isCostBetterThan(c1, c2) && !isCostBetterThan(c2, c1), as if c1 is not better than c2, and c2 is not better than c1, then they are equal. | |
virtual bool | isFinite (Cost cost) const |
Returns whether the cost is finite or not. | |
virtual Cost | betterCost (Cost c1, Cost c2) const |
Return the minimum cost given c1 and c2. Uses isCostBetterThan. | |
virtual Cost | controlCost (const control::Control *c, unsigned int steps) const |
Get the cost that corresponds to the motion created by a control c applied for duration steps. The default implementation uses the identityCost. | |
virtual Cost | combineCosts (Cost c1, Cost c2) const |
Get the cost that corresponds to combining the costs c1 and c2. Default implementation defines this combination as an addition. | |
virtual Cost | identityCost () const |
Get the identity cost value. The identity cost value is the cost c_i such that, for all costs c, combineCosts(c, c_i) = combineCosts(c_i, c) = c. In other words, combining a cost with the identity cost does not change the original cost. By default, a cost with the value 0.0 is returned. It's very important to override this with the proper identity value for your optimization objectives, or else optimal planners may not work. | |
virtual Cost | infiniteCost () const |
Get a cost which is greater than all other costs in this OptimizationObjective; required for use in Dijkstra/Astar. Defaults to returning the double value inf. | |
virtual Cost | initialCost (const State *s) const |
Returns a cost value corresponding to starting at a state s. No optimal planners currently support this method. Defaults to returning the objective's identity cost. | |
virtual Cost | terminalCost (const State *s) const |
Returns a cost value corresponding to a path ending at a state s. No optimal planners currently support this method. Defaults to returning the objective's identity cost. | |
virtual bool | isSymmetric () const |
Check if this objective has a symmetric cost metric, i.e. motionCost(s1, s2) = motionCost(s2, s1). Default implementation returns whether the underlying state space has symmetric interpolation. | |
virtual Cost | averageStateCost (unsigned int numStates) const |
Compute the average state cost of this objective by taking a sample of numStates states. | |
void | setCostToGoHeuristic (const CostToGoHeuristic &costToGo) |
Set the cost-to-go heuristic function for this objective. The cost-to-go heuristic is a function which returns an admissible estimate of the optimal path cost from a given state to a goal, where "admissible" means that the estimated cost is always less than the true optimal cost. | |
bool | hasCostToGoHeuristic () const |
Check if this objective has a cost-to-go heuristic function. | |
Cost | costToGo (const State *state, const Goal *goal) const |
Uses a cost-to-go heuristic to calculate an admissible estimate of the optimal cost from a given state to a given goal. If no cost-to-go heuristic has been specified with setCostToGoHeuristic(), this function just returns the identity cost, which is sure to be an admissible heuristic if there are no negative costs. | |
virtual Cost | motionCostHeuristic (const State *s1, const State *s2) const |
Defines an admissible estimate on the optimal cost on the motion between states s1 and s2. An admissible estimate always undervalues the true optimal cost of the motion. Used by some planners to speed up planning. The default implementation of this method returns this objective's identity cost, which is sure to be an admissible heuristic if there are no negative costs. | |
const SpaceInformationPtr & | getSpaceInformation () const |
Returns this objective's SpaceInformation. Needed for operators in MultiOptimizationObjective. | |
virtual InformedSamplerPtr | allocInformedStateSampler (const ProblemDefinitionPtr &probDefn, unsigned int maxNumberCalls) const |
Allocate a heuristic-sampling state generator for this cost function, defaults to a basic rejection sampling scheme when the derived class does not provide a better method. | |
virtual void | print (std::ostream &out) const |
Print information about this optimization objective. | |
Protected Attributes | |
std::vector< Component > | components_ |
List of objective/weight pairs. | |
bool | locked_ |
Whether this multiobjective is locked from further additions. | |
![]() | |
SpaceInformationPtr | si_ |
The space information for this objective. | |
std::string | description_ |
The description of this optimization objective. | |
Cost | threshold_ |
The cost threshold used for checking whether this objective has been satisfied during planning. | |
CostToGoHeuristic | costToGoFn_ |
The function used for returning admissible estimates on the optimal cost of the path between a given state and goal. | |
Friends | |
OptimizationObjectivePtr | operator+ (const OptimizationObjectivePtr &a, const OptimizationObjectivePtr &b) |
Given two optimization objectives, returns a MultiOptimizationObjective that combines the two objectives with both weights equal to 1.0. | |
OptimizationObjectivePtr | operator* (double weight, const OptimizationObjectivePtr &a) |
Given a weighing factor and an optimization objective, returns a MultiOptimizationObjective containing only this objective weighted by the given weight. | |
OptimizationObjectivePtr | operator* (const OptimizationObjectivePtr &a, double weight) |
Given a weighing factor and an optimization objective, returns a MultiOptimizationObjective containing only this objective weighted by the given weight. | |
Detailed Description
This class allows for the definition of multiobjective optimal planning problems. Objectives are added to this compound object, and motion costs are computed by taking a weighted sum of the individual objective costs.
Definition at line 282 of file OptimizationObjective.h.
Member Function Documentation
◆ motionCost()
|
overridevirtual |
The default implementation of this method is to use addition to add up all the individual objectives' motion cost values, where each individual value is scaled by its weight
Implements ompl::base::OptimizationObjective.
Definition at line 262 of file OptimizationObjective.cpp.
◆ stateCost()
|
overridevirtual |
The default implementation of this method is to use addition to add up all the individual objectives' state cost values, where each individual value is scaled by its weight
Implements ompl::base::OptimizationObjective.
Definition at line 251 of file OptimizationObjective.cpp.
The documentation for this class was generated from the following files:
- ompl/base/OptimizationObjective.h
- ompl/base/src/OptimizationObjective.cpp