Make the minimal number of connections required to ensure asymptotic optimality. More...
#include <ompl/geometric/planners/prm/ConnectionStrategy.h>
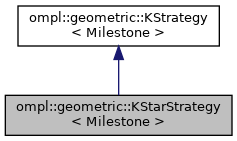
Public Types | |
using | NumNeighborsFn = std::function< unsigned int()> |
Public Member Functions | |
KStarStrategy (const NumNeighborsFn &n, const std::shared_ptr< NearestNeighbors< Milestone >> &nn, const unsigned int d=1) | |
Constructor. More... | |
const std::vector< Milestone > & | operator() (const Milestone &m) |
![]() | |
KStrategy (const unsigned int k, std::shared_ptr< NearestNeighbors< Milestone >> nn) | |
Constructor takes the maximum number of nearest neighbors to return (k) and the nearest neighbors datastruture to use (nn) | |
void | setNearestNeighbors (const std::shared_ptr< NearestNeighbors< Milestone >> &nn) |
Set the nearest neighbors datastructure to use. | |
const std::vector< Milestone > & | operator() (const Milestone &m) |
Given a milestone m, find the number of nearest neighbors connection attempts that should be made from it, according to the connection strategy. | |
unsigned int | getNumNeighbors () const |
Protected Attributes | |
const NumNeighborsFn | n_ |
Function returning the number of milestones added to the roadmap so far. | |
const double | kPRMConstant_ |
![]() | |
unsigned int | k_ |
Maximum number of nearest neighbors to attempt to connect new milestones to. | |
std::shared_ptr< NearestNeighbors< Milestone > > | nn_ |
Nearest neighbors data structure. | |
std::vector< Milestone > | neighbors_ |
Scratch space for storing k-nearest neighbors. | |
Detailed Description
template<class Milestone>
class ompl::geometric::KStarStrategy< Milestone >
Make the minimal number of connections required to ensure asymptotic optimality.
This connection strategy attempts to connect a milestone to its k-nearest neighbors where k is a function of the number of milestones that have already been added to the roadmap (n).
k(n) = kPRMConstant * log(n)
where
kPRMConstant > kStarPRMConstant = e(1 + 1/d)
and d is the number of dimensions in the state space. Note that kPRMConstant = 2e is a valid choice for any problem instance and so, if d is not provided, this value is used.
The user must provide a function that returns the value of n.
- External documentation
- S. Karaman and E. Frazzoli Sampling-based algorithms for optimal motion planning, Int. Journal of Robotics Research Volume 30, Number 7, June 2010
Definition at line 188 of file ConnectionStrategy.h.
Constructor & Destructor Documentation
◆ KStarStrategy()
|
inline |
Constructor.
- Parameters
-
n a function that returns the number of milestones that have already been added to the roadmap nn the nearest neighbors datastruture to use d the dimensionality of the state space. The default is 1, which will make kPRMConstant=2e which is valid for all problem instances.
Definition at line 201 of file ConnectionStrategy.h.
The documentation for this class was generated from the following file:
- ompl/geometric/planners/prm/ConnectionStrategy.h