ompl::NearestNeighborsFLANN< _T, _Dist > Class Template Reference
Wrapper class for nearest neighbor data structures in the FLANN library. More...
#include <ompl/datastructures/NearestNeighborsFLANN.h>
Inheritance diagram for ompl::NearestNeighborsFLANN< _T, _Dist >:
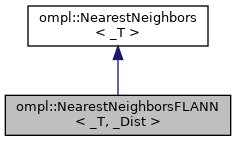
Public Member Functions | |
NearestNeighborsFLANN (std::shared_ptr< flann::IndexParams > params) | |
void | clear () override |
Clear the datastructure. | |
bool | reportsSortedResults () const override |
Return true if the solutions reported by this data structure are sorted, when calling nearestK / nearestR. | |
void | setDistanceFunction (const typename NearestNeighbors< _T >::DistanceFunction &distFun) override |
void | add (const _T &data) override |
Add an element to the datastructure. | |
void | add (const std::vector< _T > &data) override |
Add a vector of points. | |
bool | remove (const _T &data) override |
Remove an element from the datastructure. | |
_T | nearest (const _T &data) const override |
Get the nearest neighbor of a point. | |
void | nearestK (const _T &data, std::size_t k, std::vector< _T > &nbh) const override |
Return the k nearest neighbors in sorted order if searchParams_.sorted==true (the default) | |
void | nearestR (const _T &data, double radius, std::vector< _T > &nbh) const override |
Return the nearest neighbors within distance radius in sorted order if searchParams_.sorted==true (the default) | |
std::size_t | size () const override |
Get the number of elements in the datastructure. | |
void | list (std::vector< _T > &data) const override |
Get all the elements in the datastructure. | |
virtual void | setIndexParams (const std::shared_ptr< flann::IndexParams > ¶ms) |
Set the FLANN index parameters. More... | |
virtual const std::shared_ptr< flann::IndexParams > & | getIndexParams () const |
Get the FLANN parameters used to build the current index. | |
virtual void | setSearchParams (const flann::SearchParams &searchParams) |
Set the FLANN parameters to be used during nearest neighbor searches. | |
flann::SearchParams & | getSearchParams () |
Get the FLANN parameters used during nearest neighbor searches. | |
const flann::SearchParams & | getSearchParams () const |
Get the FLANN parameters used during nearest neighbor searches. | |
unsigned int | getContainerSize () const |
![]() | |
virtual void | setDistanceFunction (const DistanceFunction &distFun) |
Set the distance function to use. | |
const DistanceFunction & | getDistanceFunction () const |
Get the distance function used. | |
Protected Member Functions | |
void | createIndex (const flann::Matrix< _T > &mat) |
Internal function to construct nearest neighbor data structure with initial elements stored in mat. | |
void | rebuildIndex (unsigned int capacity=0) |
Rebuild the nearest neighbor data structure (necessary when changing the distance function or index parameters). | |
void | createIndex (const flann::Matrix< double > &mat) |
Protected Attributes | |
std::vector< _T > | data_ |
vector of data stored in FLANN's index. FLANN only indexes references, so we need store the original data. | |
flann::Index< _Dist > * | index_ |
The FLANN index (the actual index type depends on params_). | |
std::shared_ptr< flann::IndexParams > | params_ |
The FLANN index parameters. This contains both the type of index and the parameters for that type. | |
flann::SearchParams | searchParams_ |
The parameters used to seach for nearest neighbors. | |
unsigned int | dimension_ |
If each element has an array-like structure that is exposed to FLANN, then the dimension_ needs to be set to the length of this array. | |
![]() | |
DistanceFunction | distFun_ |
The used distance function. | |
Additional Inherited Members | |
![]() | |
using | DistanceFunction = std::function< double(const _T &, const _T &)> |
The definition of a distance function. | |
Detailed Description
template<typename _T, typename _Dist = FLANNDistance<_T>>
class ompl::NearestNeighborsFLANN< _T, _Dist >
Wrapper class for nearest neighbor data structures in the FLANN library.
See: M. Muja and D.G. Lowe, "Fast Approximate Nearest Neighbors with Automatic Algorithm Configuration", in International Conference on Computer Vision Theory and Applications (VISAPP'09), 2009. https://github.com/mariusmuja/flann
Definition at line 119 of file NearestNeighborsFLANN.h.
Member Function Documentation
◆ setIndexParams()
template<typename _T , typename _Dist = FLANNDistance<_T>>
|
inlinevirtual |
Set the FLANN index parameters.
The parameters determine the type of nearest neighbor data structure to be constructed.
Definition at line 273 of file NearestNeighborsFLANN.h.
The documentation for this class was generated from the following file:
- ompl/datastructures/NearestNeighborsFLANN.h