Classes |
Public Types |
Public Member Functions |
Protected Types |
Protected Member Functions |
Protected Attributes |
List of all members
ompl::Grid< _T > Class Template Reference
Representation of a simple grid. More...
#include <ompl/datastructures/Grid.h>
Inheritance diagram for ompl::Grid< _T >:
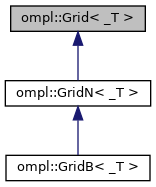
Classes | |
struct | Cell |
Definition of a cell in this grid. More... | |
struct | EqualCoordPtr |
Equality operator for coordinate pointers. More... | |
struct | HashFunCoordPtr |
struct | SortComponents |
Helper to sort components by size. More... | |
Public Types | |
using | Coord = Eigen::VectorXi |
Definition of a coordinate within this grid. | |
using | CellArray = std::vector< Cell * > |
The datatype for arrays of cells. | |
using | iterator = typename CoordHash::const_iterator |
We only allow const iterators. | |
Public Member Functions | |
Grid (unsigned int dimension) | |
The constructor takes the dimension of the grid as argument. | |
virtual | ~Grid () |
Destructor. | |
virtual void | clear () |
Clear all cells in the grid. | |
unsigned int | getDimension () const |
Return the dimension of the grid. | |
void | setDimension (unsigned int dimension) |
bool | has (const Coord &coord) const |
Check if a cell exists at the specified coordinate. | |
Cell * | getCell (const Coord &coord) const |
Get the cell at a specified coordinate. | |
void | neighbors (const Cell *cell, CellArray &list) const |
Get the list of neighbors for a given cell. | |
void | neighbors (const Coord &coord, CellArray &list) const |
Get the list of neighbors for a given coordinate. | |
void | neighbors (Coord &coord, CellArray &list) const |
Get the list of neighbors for a given coordinate. | |
std::vector< std::vector< Cell * > > | components () const |
Get the connected components formed by the cells in this grid (based on neighboring relation) | |
virtual Cell * | createCell (const Coord &coord, CellArray *nbh=nullptr) |
virtual bool | remove (Cell *cell) |
virtual void | add (Cell *cell) |
Add an instantiated cell to the grid. | |
virtual void | destroyCell (Cell *cell) const |
Clear the memory occupied by a cell; do not call this function unless remove() was called first. | |
void | getContent (std::vector< _T > &content) const |
Get the data stored in the cells we are aware of. | |
void | getCoordinates (std::vector< Coord * > &coords) const |
Get the set of coordinates where there are cells. | |
void | getCells (CellArray &cells) const |
Get the set of instantiated cells in the grid. | |
void | printCoord (Coord &coord, std::ostream &out=std::cout) const |
Print the value of a coordinate to a stream. | |
bool | empty () const |
Check if the grid is empty. | |
unsigned int | size () const |
Check the size of the grid. | |
virtual void | status (std::ostream &out=std::cout) const |
Print information about the data in this grid structure. | |
iterator | begin () const |
Return the begin() iterator for the grid. | |
iterator | end () const |
Return the end() iterator for the grid. | |
Protected Types | |
using | CoordHash = std::unordered_map< Coord *, Cell *, HashFunCoordPtr, EqualCoordPtr > |
Define the datatype for the used hash structure. | |
Protected Member Functions | |
void | freeMemory () |
Free the allocated memory. | |
Protected Attributes | |
unsigned int | dimension_ |
The dimension of the grid. | |
unsigned int | maxNeighbors_ |
The maximum number of neighbors a cell can have (2 * dimension) | |
CoordHash | hash_ |
The hash holding the cells. | |
Detailed Description
template<typename _T>
class ompl::Grid< _T >
Representation of a simple grid.
Member Function Documentation
◆ createCell()
template<typename _T >
|
inlinevirtual |
Instantiate a new cell at given coordinates; optionally Return the list of future neighbors. Note: this call only creates the cell, but does not add it to the grid. It however updates the neighbor count for neighboring cells
Reimplemented in ompl::GridB< _T, LessThanExternal, LessThanInternal >, and ompl::GridB< CellData *, OrderCellsByImportance >.
◆ remove()
template<typename _T >
|
inlinevirtual |
◆ setDimension()
template<typename _T >
|
inline |
The documentation for this class was generated from the following file:
- ompl/datastructures/Grid.h