Object containing planner generated vertex and edge data. It is assumed that all vertices are unique, and only a single directed edge connects two vertices. More...
#include <ompl/base/PlannerData.h>
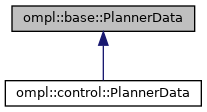
Classes | |
class | Graph |
Wrapper class for the Boost.Graph representation of the PlannerData. This class inherits from a boost::adjacency_list Graph structure. More... | |
Public Member Functions | |
PlannerData (const PlannerData &)=delete | |
PlannerData & | operator= (const PlannerData &)=delete |
PlannerData (SpaceInformationPtr si) | |
Constructor. Accepts a SpaceInformationPtr for the space planned in. | |
virtual | ~PlannerData () |
Destructor. | |
const SpaceInformationPtr & | getSpaceInformation () const |
Return the instance of SpaceInformation used in this PlannerData. | |
virtual bool | hasControls () const |
Indicate whether any information about controls (ompl::control::Control) is stored in this instance. | |
PlannerData construction | |
unsigned int | addVertex (const PlannerDataVertex &st) |
Adds the given vertex to the graph data. The vertex index is returned. Duplicates are not added. If a vertex is duplicated, the index of the existing vertex is returned instead. Indexes are volatile and may change after adding/removing a subsequent vertex. | |
unsigned int | addStartVertex (const PlannerDataVertex &v) |
Adds the given vertex to the graph data, and marks it as a start vertex. The vertex index is returned. Duplicates are not added. If a vertex is duplicated, the index of the existing vertex is returned instead. Indexes are volatile and may change after adding/removing a subsequent vertex. | |
unsigned int | addGoalVertex (const PlannerDataVertex &v) |
Adds the given vertex to the graph data, and marks it as a start vertex. The vertex index is returned. Duplicates are not added. If a vertex is duplicated, the index of the existing vertex is returned instead. Indexes are volatile and may change after adding/removing a subsequent vertex. | |
bool | markStartState (const State *st) |
Mark the given state as a start vertex. If the given state does not exist in a vertex, false is returned. | |
bool | markGoalState (const State *st) |
Mark the given state as a goal vertex. If the given state does not exist in a vertex, false is returned. | |
bool | tagState (const State *st, int tag) |
Set the integer tag associated with the given state. If the given state does not exist in a vertex, false is returned. | |
virtual bool | removeVertex (const PlannerDataVertex &st) |
Removes the vertex associated with the given data. If the vertex does not exist, false is returned. This method has O(n) complexity in the number of vertices. | |
virtual bool | removeVertex (unsigned int vIndex) |
Removes the vertex with the given index. If the index is out of range, false is returned. This method has O(n) complexity in the number of vertices. | |
virtual bool | addEdge (unsigned int v1, unsigned int v2, const PlannerDataEdge &edge=PlannerDataEdge(), Cost weight=Cost(1.0)) |
Adds a directed edge between the given vertex indexes. An optional edge structure and weight can be supplied. Success is returned. | |
virtual bool | addEdge (const PlannerDataVertex &v1, const PlannerDataVertex &v2, const PlannerDataEdge &edge=PlannerDataEdge(), Cost weight=Cost(1.0)) |
Adds a directed edge between the given vertex indexes. The vertices are added to the data if they are not already in the structure. An optional edge structure and weight can also be supplied. Success is returned. | |
virtual bool | removeEdge (unsigned int v1, unsigned int v2) |
Removes the edge between vertex indexes v1 and v2. Success is returned. | |
virtual bool | removeEdge (const PlannerDataVertex &v1, const PlannerDataVertex &v2) |
Removes the edge between the vertices associated with the given vertex data. Success is returned. | |
virtual void | clear () |
Clears the entire data structure. | |
virtual void | decoupleFromPlanner () |
Creates a deep copy of the states contained in the vertices of this PlannerData structure so that when the planner that created this instance goes out of scope, all data remains intact. More... | |
PlannerData Properties | |
unsigned int | numEdges () const |
Retrieve the number of edges in this structure. | |
unsigned int | numVertices () const |
Retrieve the number of vertices in this structure. | |
unsigned int | numStartVertices () const |
Returns the number of start vertices. | |
unsigned int | numGoalVertices () const |
Returns the number of goal vertices. | |
PlannerData vertex lookup | |
bool | vertexExists (const PlannerDataVertex &v) const |
Check whether a vertex exists with the given vertex data. | |
const PlannerDataVertex & | getVertex (unsigned int index) const |
Retrieve a reference to the vertex object with the given index. If this vertex does not exist, NO_VERTEX is returned. | |
PlannerDataVertex & | getVertex (unsigned int index) |
Retrieve a reference to the vertex object with the given index. If this vertex does not exist, NO_VERTEX is returned. | |
const PlannerDataVertex & | getStartVertex (unsigned int i) const |
Retrieve a reference to the ith start vertex object. If i is greater than the number of start vertices, NO_VERTEX is returned. | |
PlannerDataVertex & | getStartVertex (unsigned int i) |
Retrieve a reference to the ith start vertex object. If i is greater than the number of start vertices, NO_VERTEX is returned. | |
const PlannerDataVertex & | getGoalVertex (unsigned int i) const |
Retrieve a reference to the ith goal vertex object. If i is greater than the number of goal vertices, NO_VERTEX is returned. | |
PlannerDataVertex & | getGoalVertex (unsigned int i) |
Retrieve a reference to the ith goal vertex object. If i is greater than the number of goal vertices, NO_VERTEX is returned. | |
unsigned int | getStartIndex (unsigned int i) const |
Returns the index of the ith start state. INVALID_INDEX is returned if i is out of range. Indexes are volatile and may change after adding/removing a vertex. | |
unsigned int | getGoalIndex (unsigned int i) const |
Returns the index of the ith goal state. INVALID_INDEX is returned if i is out of range Indexes are volatile and may change after adding/removing a vertex. | |
bool | isStartVertex (unsigned int index) const |
Returns true if the given vertex index is marked as a start vertex. | |
bool | isGoalVertex (unsigned int index) const |
Returns true if the given vertex index is marked as a goal vertex. | |
unsigned int | vertexIndex (const PlannerDataVertex &v) const |
Return the index for the vertex associated with the given data. INVALID_INDEX is returned if this vertex does not exist. Indexes are volatile and may change after adding/removing a vertex. | |
PlannerData edge lookup | |
bool | edgeExists (unsigned int v1, unsigned int v2) const |
Check whether an edge between vertex index v1 and index v2 exists. | |
const PlannerDataEdge & | getEdge (unsigned int v1, unsigned int v2) const |
Retrieve a reference to the edge object connecting vertices with indexes v1 and v2. If this edge does not exist, NO_EDGE is returned. | |
PlannerDataEdge & | getEdge (unsigned int v1, unsigned int v2) |
Retrieve a reference to the edge object connecting vertices with indexes v1 and v2. If this edge does not exist, NO_EDGE is returned. | |
unsigned int | getEdges (unsigned int v, std::vector< unsigned int > &edgeList) const |
Returns a list of the vertex indexes directly connected to vertex with index v (outgoing edges). The number of outgoing edges from v is returned. | |
unsigned int | getEdges (unsigned int v, std::map< unsigned int, const PlannerDataEdge * > &edgeMap) const |
Returns a map of outgoing edges from vertex with index v. Key = vertex index, value = edge structure. The number of outgoing edges from v is returned. | |
unsigned int | getIncomingEdges (unsigned int v, std::vector< unsigned int > &edgeList) const |
Returns a list of vertices with outgoing edges to the vertex with index v. The number of edges connecting to v is returned. | |
unsigned int | getIncomingEdges (unsigned int v, std::map< unsigned int, const PlannerDataEdge * > &edgeMap) const |
Returns a map of incoming edges to the vertex with index v (i.e. if there is an edge from w to v, w and the edge structure will be in the map.) Key = vertex index, value = edge structure. The number of incoming edges to v is returned. | |
bool | getEdgeWeight (unsigned int v1, unsigned int v2, Cost *weight) const |
Returns the weight of the edge between the given vertex indices. If there exists an edge between v1 and \v2, the edge weight is placed in the out-variable weight. Otherwise, this function returns false. | |
bool | setEdgeWeight (unsigned int v1, unsigned int v2, Cost weight) |
Sets the weight of the edge between the given vertex indices. If an edge between v1 and v2 does not exist, this function returns false. | |
void | computeEdgeWeights (const OptimizationObjective &opt) |
Computes the weight for all edges given the OptimizationObjective opt. | |
void | computeEdgeWeights () |
Computes all edge weights using state space distance (i.e. getSpaceInformation()->distance()) | |
Output methods | |
void | printGraphviz (std::ostream &out=std::cout) const |
Writes a Graphviz dot file of this structure to the given stream. | |
void | printGraphML (std::ostream &out=std::cout) const |
Writes a GraphML file of this structure to the given stream. | |
void | printPLY (std::ostream &out, bool asIs=false) const |
Write a mesh of the planner graph to a stream. Insert additional vertices if asIs == true. | |
Advanced graph extraction | |
void | extractMinimumSpanningTree (unsigned int v, const OptimizationObjective &opt, PlannerData &mst) const |
Extracts the minimum spanning tree of the data rooted at the vertex with index v. The minimum spanning tree is saved into mst. O(|E| log |V|) complexity. | |
void | extractReachable (unsigned int v, PlannerData &data) const |
Extracts the subset of PlannerData reachable from the vertex with index v. For tree structures, this will be the sub-tree rooted at v. The reachable set is saved into data. | |
StateStoragePtr | extractStateStorage () const |
Extract a ompl::base::GraphStateStorage object from this PlannerData. Memory for states is copied (the resulting ompl::base::StateStorage is independent from this PlannerData) | |
Graph & | toBoostGraph () |
Extract a Boost.Graph object from this PlannerData. More... | |
const Graph & | toBoostGraph () const |
Extract a Boost.Graph object from this PlannerData. More... | |
Public Attributes | |
std::map< std::string, std::string > | properties |
Any extra properties (key-value pairs) the planner can set. | |
Static Public Attributes | |
static const PlannerDataEdge | NO_EDGE = ompl::base::PlannerDataEdge() |
Representation for a non-existant edge. | |
static const PlannerDataVertex | NO_VERTEX = ompl::base::PlannerDataVertex(nullptr) |
Representation for a non-existant vertex. | |
static const unsigned int | INVALID_INDEX = std::numeric_limits<unsigned int>::max() |
Representation of an invalid vertex index. | |
Protected Attributes | |
std::map< const State *, unsigned int > | stateIndexMap_ |
A mapping of states to vertex indexes. For fast lookup of vertex index. | |
std::vector< unsigned int > | startVertexIndices_ |
A mutable listing of the vertices marked as start states. Stored in sorted order. | |
std::vector< unsigned int > | goalVertexIndices_ |
A mutable listing of the vertices marked as goal states. Stored in sorted order. | |
SpaceInformationPtr | si_ |
The space information instance for this data. | |
std::set< State * > | decoupledStates_ |
A list of states that are allocated during the decoupleFromPlanner method. These states are freed by PlannerData in the destructor. | |
Detailed Description
Object containing planner generated vertex and edge data. It is assumed that all vertices are unique, and only a single directed edge connects two vertices.
- Note
- The storage for states this class maintains belongs to the planner instance that filled the data (by default; see PlannerData::decoupleFromPlanner())
Definition at line 238 of file PlannerData.h.
Member Function Documentation
◆ decoupleFromPlanner()
|
virtual |
Creates a deep copy of the states contained in the vertices of this PlannerData structure so that when the planner that created this instance goes out of scope, all data remains intact.
- Remarks
- Shallow state pointers inside of the PlannerDataVertex objects already in this PlannerData will be replaced with clones which are scoped to this PlannerData object. A subsequent call to this method is necessary after any other vertices are added to ensure that this PlannerData instance is fully decoupled.
Reimplemented in ompl::control::PlannerData.
Definition at line 80 of file PlannerData.cpp.
◆ toBoostGraph() [1/2]
const ompl::base::PlannerData::Graph & ompl::base::PlannerData::toBoostGraph | ( | ) |
Extract a Boost.Graph object from this PlannerData.
- Remarks
- Use of this method requires inclusion of PlannerDataGraph.h The object returned can be used safely for all read-only purposes in Boost. Adding or removing vertices and edges should be performed by using the respective method in PlannerData to ensure proper memory management. Manipulating the graph directly will result in undefined behavior with this class.
Definition at line 744 of file PlannerData.cpp.
◆ toBoostGraph() [2/2]
const Graph& ompl::base::PlannerData::toBoostGraph | ( | ) | const |
Extract a Boost.Graph object from this PlannerData.
- Remarks
- Use of this method requires inclusion of PlannerDataGraph.h The object returned can be used safely for all read-only purposes in Boost. Adding or removing vertices and edges should be performed by using the respective method in PlannerData to ensure proper memory management. Manipulating the graph directly will result in undefined behavior with this class.
The documentation for this class was generated from the following files:
- ompl/base/PlannerData.h
- ompl/base/src/PlannerData.cpp