Definition of a problem to be solved. This includes the start state(s) for the system and a goal specification. Will contain solutions, if found.
More...
#include <ompl/base/ProblemDefinition.h>
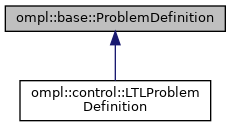
Public Member Functions | |
ProblemDefinition (const ProblemDefinition &)=delete | |
ProblemDefinition & | operator= (const ProblemDefinition &)=delete |
ProblemDefinition (SpaceInformationPtr si) | |
Create a problem definition given the SpaceInformation it is part of. | |
ProblemDefinitionPtr | clone () const |
Return a copy of the problem definition. More... | |
const SpaceInformationPtr & | getSpaceInformation () const |
Get the space information this problem definition is for. | |
void | addStartState (const State *state) |
Add a start state. The state is copied. | |
void | addStartState (const ScopedState<> &state) |
Add a start state. The state is copied. More... | |
bool | hasStartState (const State *state, unsigned int *startIndex=nullptr) const |
Check whether a specified starting state is already included in the problem definition and optionally return the index of that starting state. | |
void | clearStartStates () |
Clear all start states (memory is freed) | |
unsigned int | getStartStateCount () const |
Returns the number of start states. | |
const State * | getStartState (unsigned int index) const |
Returns a specific start state. | |
State * | getStartState (unsigned int index) |
Returns a specific start state. More... | |
void | setGoal (const GoalPtr &goal) |
Set the goal. | |
void | clearGoal () |
Clear the goal. Memory is freed. | |
const GoalPtr & | getGoal () const |
Return the current goal. | |
void | getInputStates (std::vector< const State * > &states) const |
Get all the input states. This includes start states and states that are part of goal regions that can be casted as ompl::base::GoalState or ompl::base::GoalStates. | |
void | setStartAndGoalStates (const State *start, const State *goal, double threshold=std::numeric_limits< double >::epsilon()) |
In the simplest case possible, we have a single starting state and a single goal state. More... | |
void | setGoalState (const State *goal, double threshold=std::numeric_limits< double >::epsilon()) |
A simple form of setting the goal. This is called by setStartAndGoalStates(). A more general form is setGoal() | |
void | setStartAndGoalStates (const ScopedState<> &start, const ScopedState<> &goal, const double threshold=std::numeric_limits< double >::epsilon()) |
In the simplest case possible, we have a single starting state and a single goal state. More... | |
void | setGoalState (const ScopedState<> &goal, const double threshold=std::numeric_limits< double >::epsilon()) |
A simple form of setting the goal. This is called by setStartAndGoalStates(). A more general form is setGoal() More... | |
bool | hasOptimizationObjective () const |
Check if an optimization objective was defined for planning | |
const OptimizationObjectivePtr & | getOptimizationObjective () const |
Get the optimization objective to be considered during planning. | |
void | setOptimizationObjective (const OptimizationObjectivePtr &optimizationObjective) |
Set the optimization objective to be considered during planning. | |
const ReportIntermediateSolutionFn & | getIntermediateSolutionCallback () const |
When this function returns a valid function pointer, that function should be called by planners that compute intermediate solutions every time a better solution is found. | |
void | setIntermediateSolutionCallback (const ReportIntermediateSolutionFn &callback) |
Set the callback to be called by planners that can compute intermediate solutions. | |
bool | isTrivial (unsigned int *startIndex=nullptr, double *distance=nullptr) const |
A problem is trivial if a given starting state already in the goal region, so we need no motion planning. startID will be set to the index of the starting state that satisfies the goal. The distance to the goal can optionally be returned as well. | |
PathPtr | isStraightLinePathValid () const |
Check if a straight line path is valid. If it is, return an instance of a path that represents the straight line. More... | |
bool | fixInvalidInputStates (double distStart, double distGoal, unsigned int attempts) |
Many times the start or goal state will barely touch an obstacle. In this case, we may want to automatically find a nearby state that is valid so motion planning can be performed. This function enables this behaviour. The allowed distance for both start and goal states is specified. The number of attempts is also specified. Returns true if all states are valid after completion. | |
bool | hasSolution () const |
Returns true if a solution path has been found (could be approximate) | |
bool | hasExactSolution () const |
Returns true if an exact solution path has been found. Specifically returns hasSolution && !hasApproximateSolution() | |
bool | hasApproximateSolution () const |
Return true if the top found solution is approximate (does not actually reach the desired goal, but hopefully is closer to it) | |
double | getSolutionDifference () const |
Get the distance to the desired goal for the top solution. Return -1.0 if there are no solutions available. | |
bool | hasOptimizedSolution () const |
Return true if the top found solution is optimized (satisfies the specified optimization objective) | |
PathPtr | getSolutionPath () const |
Return the top solution path, if one is found. The top path is a shortest path that was found, preference being given to solutions that are not approximate. More... | |
bool | getSolution (PlannerSolution &solution) const |
Return true if a top solution is found, with the top solution passed by reference in the function header The top path is a shortest path that was found, preference being given to solutions that are not approximate. This will need to be casted into the specialization computed by the planner. | |
void | addSolutionPath (const PathPtr &path, bool approximate=false, double difference=-1.0, const std::string &plannerName="Unknown") const |
Add a solution path in a thread-safe manner. Multiple solutions can be set for a goal. If a solution does not reach the desired goal it is considered approximate. Optionally, the distance between the desired goal and the one actually achieved is set by difference. Optionally, the name of the planner that generated the solution. | |
void | addSolutionPath (const PlannerSolution &sol) const |
Add a solution path in a thread-safe manner. Multiple solutions can be set for a goal. | |
std::size_t | getSolutionCount () const |
Get the number of solutions already found. | |
std::vector< PlannerSolution > | getSolutions () const |
Get all the solution paths available for this goal. | |
void | clearSolutionPaths () const |
Forget the solution paths (thread safe). Memory is freed. | |
bool | hasSolutionNonExistenceProof () const |
Returns true if the problem definition has a proof of non existence for a solution. | |
void | clearSolutionNonExistenceProof () |
Removes any existing instance of SolutionNonExistenceProof. | |
const SolutionNonExistenceProofPtr & | getSolutionNonExistenceProof () const |
Retrieve a pointer to the SolutionNonExistenceProof instance for this problem definition. | |
void | setSolutionNonExistenceProof (const SolutionNonExistenceProofPtr &nonExistenceProof) |
Set the instance of SolutionNonExistenceProof for this problem definition. | |
void | print (std::ostream &out=std::cout) const |
Print information about the start and goal states and the optimization objective. | |
Protected Member Functions | |
bool | fixInvalidInputState (State *state, double dist, bool start, unsigned int attempts) |
Helper function for fixInvalidInputStates(). Attempts to fix an individual state. | |
Protected Attributes | |
SpaceInformationPtr | si_ |
The space information this problem definition is for. | |
std::vector< State * > | startStates_ |
The set of start states. | |
GoalPtr | goal_ |
The goal representation. | |
SolutionNonExistenceProofPtr | nonExistenceProof_ |
A Representation of a proof of non-existence of a solution for this problem definition. | |
OptimizationObjectivePtr | optimizationObjective_ |
The objective to be optimized while solving the planning problem. | |
ReportIntermediateSolutionFn | intermediateSolutionCallback_ |
Callback function which is called when a new intermediate solution has been found. | |
Detailed Description
Definition of a problem to be solved. This includes the start state(s) for the system and a goal specification. Will contain solutions, if found.
Definition at line 216 of file ProblemDefinition.h.
Member Function Documentation
◆ addStartState()
|
inline |
Add a start state. The state is copied.
Definition at line 252 of file ProblemDefinition.h.
◆ clone()
ompl::base::ProblemDefinitionPtr ompl::base::ProblemDefinition::clone | ( | ) | const |
Return a copy of the problem definition.
A deep copy is made of the start and goal states. A shallow copy is made of shared ptrs. The set of solutions paths and the intermediate solution callback function are not copied.
Definition at line 168 of file ProblemDefinition.cpp.
◆ getSolutionPath()
ompl::base::PathPtr ompl::base::ProblemDefinition::getSolutionPath | ( | ) | const |
Return the top solution path, if one is found. The top path is a shortest path that was found, preference being given to solutions that are not approximate.
This will need to be casted into the specialization computed by the planner
Definition at line 414 of file ProblemDefinition.cpp.
◆ getStartState()
|
inline |
Returns a specific start state.
Definition at line 283 of file ProblemDefinition.h.
◆ isStraightLinePathValid()
ompl::base::PathPtr ompl::base::ProblemDefinition::isStraightLinePathValid | ( | ) | const |
Check if a straight line path is valid. If it is, return an instance of a path that represents the straight line.
- Note
- When planning under geometric constraints, this works only if the goal region can be sampled. If the goal region cannot be sampled, this call is equivalent to calling isTrivial()
- When planning under differential constraints, the system is propagated forward in time using the null control.
Definition at line 290 of file ProblemDefinition.cpp.
◆ setGoalState()
|
inline |
A simple form of setting the goal. This is called by setStartAndGoalStates(). A more general form is setGoal()
Definition at line 334 of file ProblemDefinition.h.
◆ setStartAndGoalStates() [1/2]
|
inline |
In the simplest case possible, we have a single starting state and a single goal state.
This function simply configures the problem definition using these states (performs the needed calls to addStartState(), creates an instance of ompl::base::GoalState and calls setGoal() on it.
Definition at line 327 of file ProblemDefinition.h.
◆ setStartAndGoalStates() [2/2]
void ompl::base::ProblemDefinition::setStartAndGoalStates | ( | const State * | start, |
const State * | goal, | ||
double | threshold = std::numeric_limits<double>::epsilon() |
||
) |
In the simplest case possible, we have a single starting state and a single goal state.
This function simply configures the problem definition using these states (performs the needed calls to addStartState(), creates an instance of ompl::base::GoalState and calls setGoal() on it.
Definition at line 181 of file ProblemDefinition.cpp.
The documentation for this class was generated from the following files:
- ompl/base/ProblemDefinition.h
- ompl/base/src/ProblemDefinition.cpp